As your application grows, ensuring it remains operational is crucial. Let's explore how you can add a health check endpoint to your Next.js app, providing you with peace of mind and proactive monitoring capabilities.
Why would we need a health check endpoint?
Creating a health check endpoint is straightforward and doesn't require extensive technical expertise. By monitoring this endpoint, you can receive timely alerts when your application encounters issues.
Key reasons for implementing a health check endpoint include:
- SLA (Service Level Agreement) — You're often obligated to maintain a specific uptime percentage as outlined in your SLA.
- Financial Impact — Downtime can translate to substantial financial losses, especially in certain industries where even a minute of outage can be costly.
- Reputation Management — Frequent downtime incidents can severely damage your business's reputation and erode customer trust.
Code
Create a new file called health.js
in your pages/api
directory:
export default function handler(req, res) {
res.status(200).json({ status: 'OK' });
}
This creates a simple endpoint that returns a 200 status code with a JSON response indicating your app is healthy.
Let's enhance this further by providing more detailed information:
export default function handler(req, res) {
res.status(200).json({
status: 'OK',
uptime: process.uptime(),
timestamp: new Date().toISOString(),
});
}
If your app uses a database (like Prisma), you can add a connectivity check:
import prisma from '@/lib/prisma';
export default async function handler(req, res) {
try {
await prisma.$queryRaw`SELECT 1`;
res.status(200).json({
status: 'OK',
database: 'Connected',
uptime: process.uptime(),
timestamp: new Date().toISOString(),
});
} catch (error) {
res.status(500).json({
status: 'ERROR',
database: 'Disconnected',
error: error.message,
});
}
}
For additional security, you might want to add basic authentication:
export default function handler(req, res) {
if (req.headers['x-api-key'] !== process.env.HEALTHCHECK_KEY) {
return res.status(401).json({ error: 'Unauthorized' });
}
res.status(200).json({
status: 'OK',
uptime: process.uptime(),
timestamp: new Date().toISOString(),
});
}
Automate the checks
With your new health check endpoint in place, you can now proactively monitor your application's status and receive timely alerts when issues arise. To effectively track uptime, response time, and receive prompt notifications of any failures, consider using a dedicated uptime monitoring service like Hyperping. Create an account, and you'll be guided through the process of setting up your first monitor.
By simply adding your new health check endpoint to Hyperping, you can continuously monitor its availability without any complex configuration. After a few minutes, you'll gain valuable insights into your application's uptime and response time across various geographic regions (London, Amsterdam, Toronto, San Francisco, and more), along with historical data.
Furthermore, Hyperping seamlessly integrates with popular alerting tools like Slack, PagerDuty, and SMS, allowing you to notify your team instantly of any detected issues.
Conclusion
Setting up a health check endpoint in your Next.js application is a straightforward process that provides invaluable peace of mind. By proactively monitoring your application's health, you can ensure greater stability and reliability.
Start monitoring your Next.js application with Hyperping and get 14 days of free monitoring to ensure your application stays healthy and available.
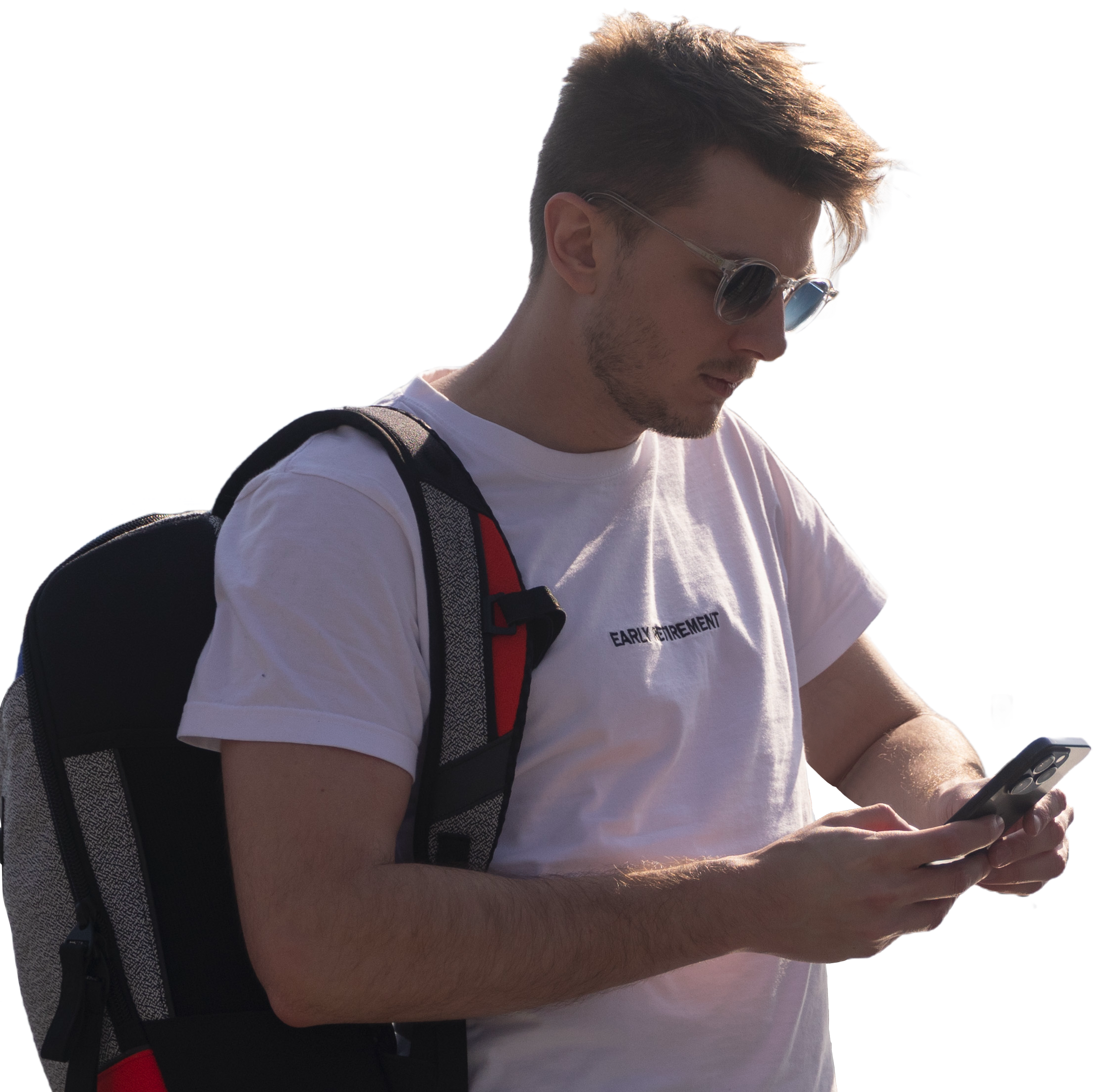
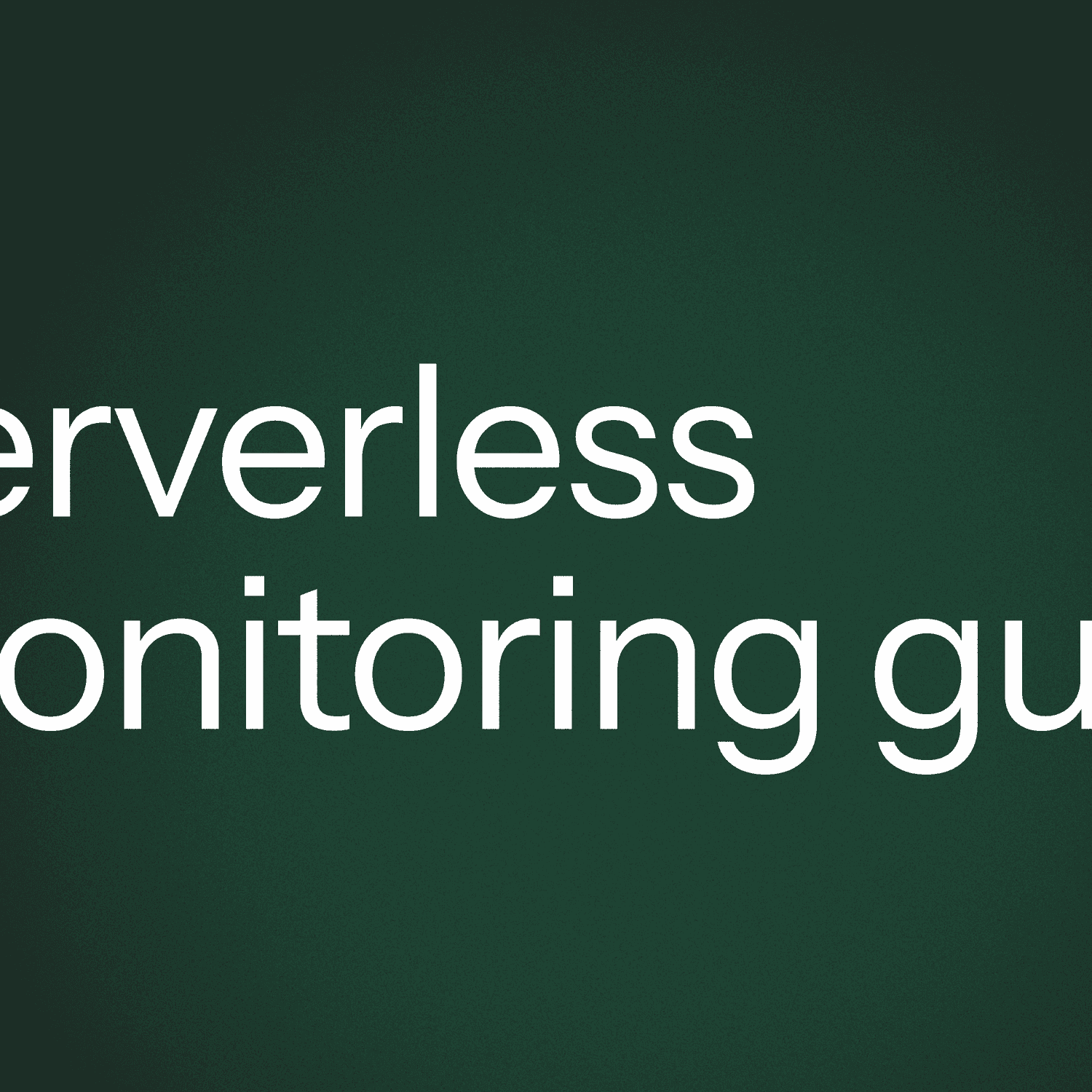
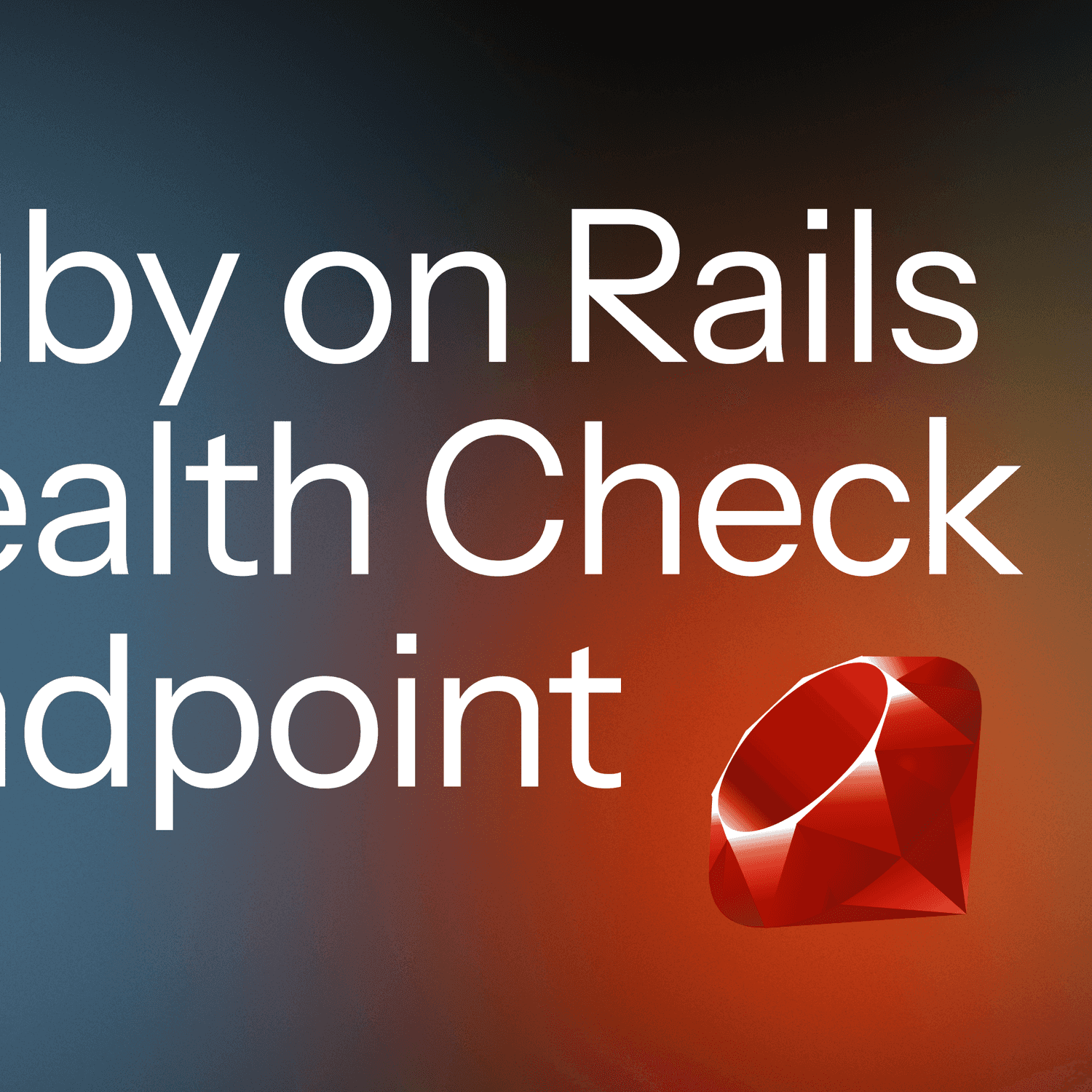